Python
Learn how to submit jobs to the super.AI platform using Python.
The super.AI API provides programmatic access to super.AI. In this guide, you will get set up by submitting some jobs using Python and viewing them in your dashboard.
cURL, Python, and the command-line interface (CLI)
This guide uses Python, but you can also interact with our API using cURL or the super.AI CLI.
If you're new to APIs, take a look at our Introduction to REST APIs to learn the the basics.
Python and environment management
This guide assumes you have Python 3.6 or later installed on your machine and know how to manage environments. You can run
python --version
in your terminal to check which version you have installed. If you’re new to Python, we recommend taking a look at miniconda, which is a lightweight installer for Python, pip, and Conda, an open-source environment management system.
There are five steps required to submit your first job to super.AI using Python:
- Install the super.AI API client Python package so you have the tools needed to interact with our API
- Obtain your API key so super.AI can authenticate your request
- Locate your project ID to identify the project to which you are submitting the job
- Write your Python script that will trigger the API request
- Run the script and observe the response to confirm your jobs were submitted
You will need to create and set up a super.AI project before you can follow the steps below. You will need to use the image categorization project type to follow this guide.
1. Install the super.AI API client
In your terminal window, enter the following command and press enter:
$ pip install superai
You should see confirmation of a successful installation.
2. Obtain your API key
Super.AI relies on API keys to authenticate requests. Any request that does not include a valid API key will fail. Find your API key in the super.AI dashboard by hovering over the profile icon in the lower left of the screen, then heading to API keys. You can copy the key by clicking on the copy button.
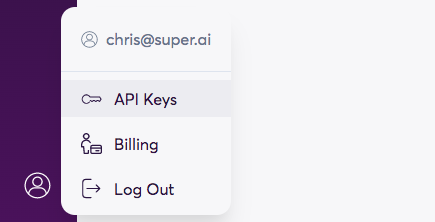
3. Locate your project ID
The project ID is essential to identify the project to which you want to submit a job.
In the super.AI dashboard, open the image categorization project that you created previously. The project ID is the universally unique identifier visible in your browser's URL bar.

You’re going to pass us the project ID as a keyword argument in your Python script, as you’ll see in the next step.
(Optional) Upload data to storage
For the purposes of this guide, we provide you with a URL for an image in the next step. If you want to upload your own images, you can upload them to your super.AI storage and generate URLs for them there. To find out more, head to our How to use data storage page.
4. Write your Python script
There are four important elements to your Python script:
- The super.AI function you call. We’re creating a job so we call the
create_jobs()
function - The project ID. You will pass this as a keyword argument into the
create_jobs()
function. - Your API key. You will pass this into the
Client()
function. - The input data for the job. This is passed as a list of dictionaries, one for each data point. Each data point’s dictionary has a key-value pair for each input. The key is the input name and the value is the value (in our case,
image_url
and the URL pointing to the file, respectively).
In the code below, you will need to make the following changes:
- Replace
{api_key}
with the API key from step 1 - Replace
{project_id}
with the project ID from step 2
import superai as ai
client = ai.Client("{api_key}")
response = client.create_jobs(
app_id="{project_id}",
inputs=[
{"image_url": "https://cdn.super.ai/cool-bulldog.jpg"},
{"image_url": "https://cdn.super.ai/hot-dog-01.jpeg"},
],
)
print(response)
Copy and paste the above code into a text editor, or download the Python file and make the necessary changes before saving.
5. Make an API request
In your terminal window, navigate to the folder where you saved your Python file, and enter the following command:
$ python getting-started.py
Once you’ve submitted this command, your terminal should display a response similar to this one:
$ {'message': '2 jobs submitted', 'truncated_inputs': False, 'batch_id': '12a34b56-cde7-8901-2345-fg6789hijk01'}
As you’ve submitted more than one image input, the data points are grouped into a batch. The batch_id
allows you to retrieve information on the batch via our API.
API request complete
Congratulations! You have successfully made your first request and created a job through the super.AI API.
You can view your submitted jobs in the super.AI dashboard by navigating to your project and taking a look at the top of the project’s work queue.
Updated almost 2 years ago
Now that you’re familiar with the basics of using Python to interact with our API, you can dive into our API reference to explore the other functions on offer.